Closures, Scope and All the Good Stuff About Javascript
. โ 5 mins read
Objectives
This article was written to help explain a topic which is an interview staple for Javascript developers. Based on the assumption that the reader is a total newbie to the topic, we will start from the very basics and incrementally build up on our knowledge. Hopefully by the end of this article you will have a solid understanding of closures.
Prerequisites
- Nodejs installed locally.
- Text editor of your choice.
Key Concepts
Variable
A variable in Javascript is an entity that is used to refer to data stored in memory. You declare a variable in your script by giving it a name, which is preceded by a mandatory keyword which can either be let, const or var and assigning it a value. Assigning an initial value is optional for any variable preceded by keyword let. All var and let variables can be reassigned values whereas const variables are immutable once declared and initialized.
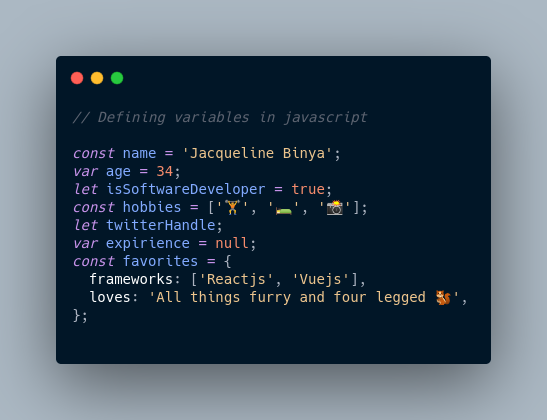
To learn more about variable declaration, initialization, data types and hoisting check out this link. It contains resources published by mozilla (MDN).
Block
A block (or compound statement in other programming languages) is used to group statements in javascript. The boundaries of a block statement is a pair of curly brackets.
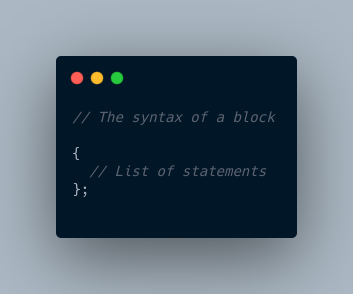
On that basis of the given definition of a block, it follows that functions, if statements, loops all have block statements.
Local and Global Variables
In Javascript variables are either declared inside a block or independent of a block. When a variable is declared inside a block it is local in reference to that block. A global variable is one whose scope is global.
What then is scope ?
In Javascript scope denotes the reach or range or accessibility of Javascript variables. So, whenever a javascript variable is declared it automatically has scope associated with it.
The scope of a variable is determined by:
- where a variable is declared within a script and,
- its type i.e let, const and var.
Types of scope
-
Global scope: globally scoped variables are accessible throughout the window object or globally within your script.
-
Block scope: block scoped variables are variables that when declared inside of a block are only accessible within that block. The block referenced to, being a generic block or a block which is part of a loop or an if statement or a function. So essentially const and let variables can either be globally scoped if declared outside of a block or block scoped when declared inside of a block. When var variables are declared in blocks which aren’t functions, their scope is global.
In the screenshot below I declared a couple of variables, I suggest you go to your text editor and create a main.js file and copy the contents of the screenshot and modify them to your liking.
Now to execute main.js file, type the command below in your terminal:
node main.js
The expected results are shown in the screenshot below:
The variables name, lastName and isSoftwareDeveloper are declared independent of a block. These are known as global variables and they have global scope.
Variables hobbies and countryOfResidence are local to a block in which they were declared but, although hobbies has global scope countryOfResidence is block scoped.
- Functional Scope: functionally scoped variables are variables that when declared inside of a function they are only accessible within that function, if the same variable was declared in any other block e.g. an if statement and it was accessible only within that block as well. Its scope is then described as being block scoped. So essentially functional scope is a special type of block scope used to describe the scope of var variables declared inside functions. A var variable is always globally scoped otherwise it is functionally scoped if declared inside of a function.
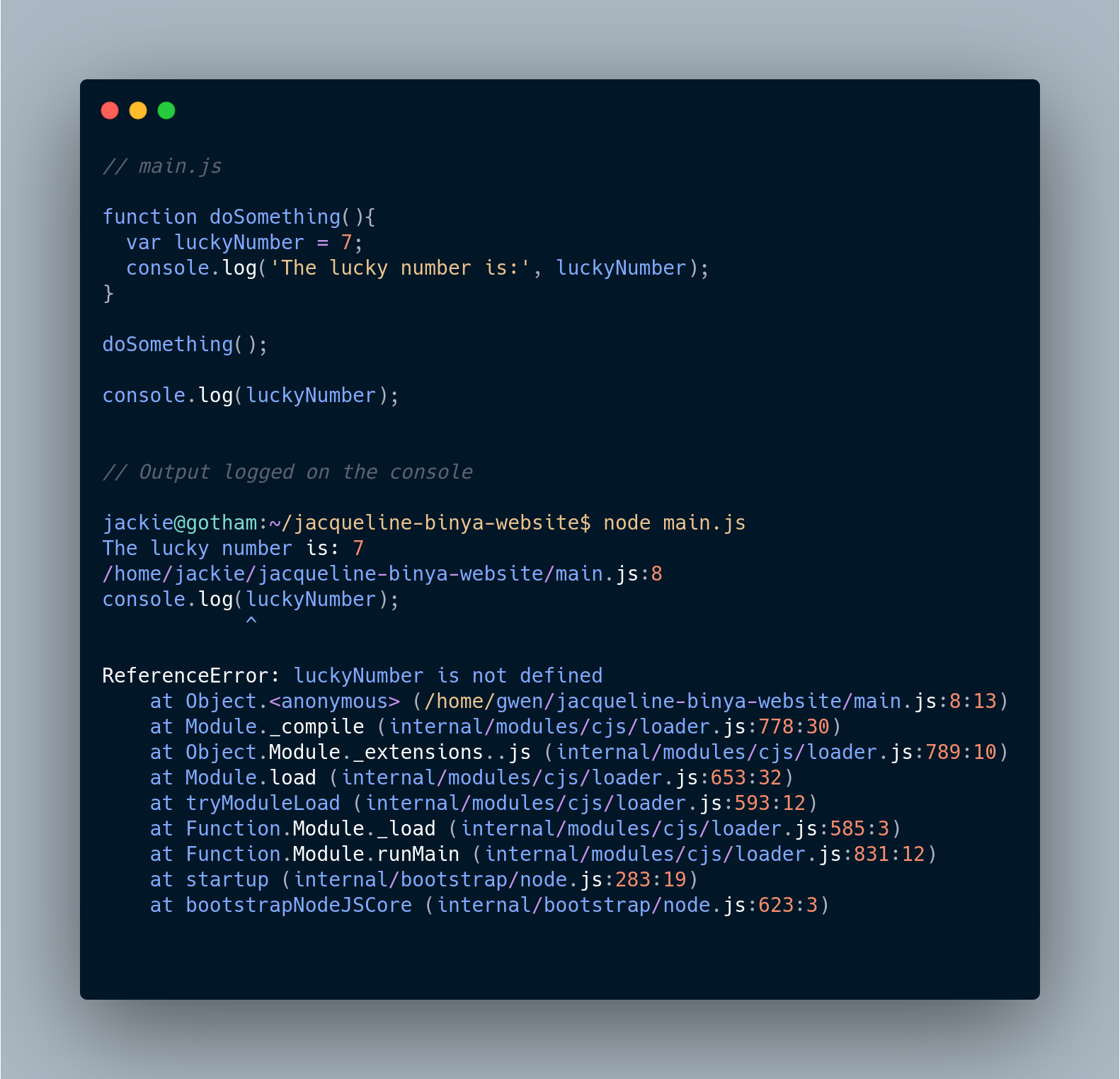
- Lexical Scope: given a scenario where we have a group of nested functions, lexical scope means that the inner functions have access to the variables and/or resources of the outer function even after the outer function is returned.
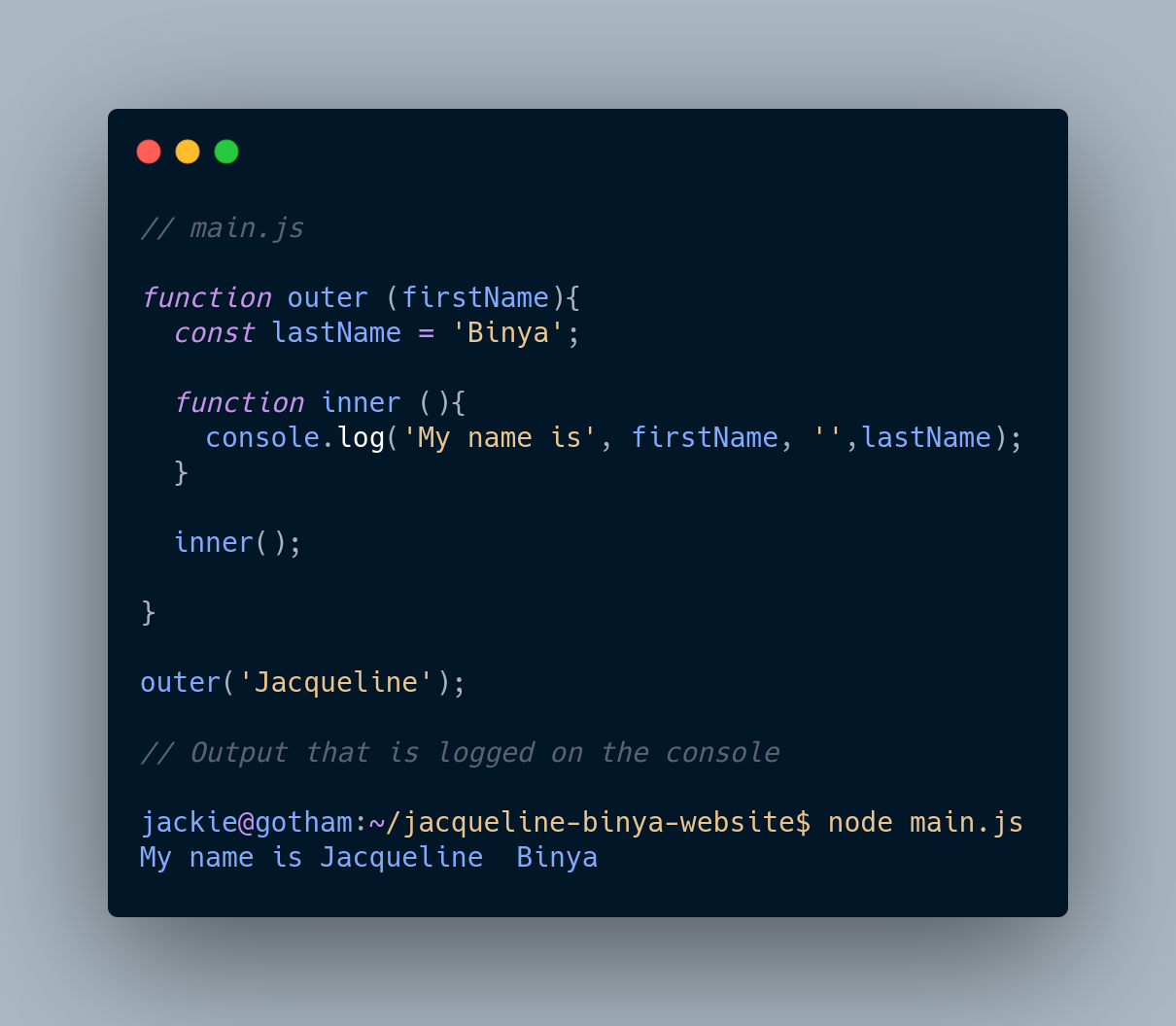
Finally, what then are closures ?
To truly understand closures we need to have a basic understanding of how the Javascript engine executes functions. So, whenever a function is invoked an execution context associated with the function is created. An execution context contains meta data about the function and all its associated variables. Upon execution of the function all the meta data associated with the function is then removed from the memory, what is often refereed to as garbage collection.
Closures are functions which have references to variables in their lexical environment.
Let’s reconsider the screenshot provided above, when the file is executed internally what happens is that, the javascript engine parses main.js line by line. When it reaches the point in the script where the outer function is invoked it creates a context associated with it. When the javascript engine eventually executes the inner function is creates an execution context associated with it as well. Now as it was verified above, the variables of the outer function are always accessible inside the inner function, even when the outer function’s execution context has been removed from memory. Let’s see how that is possible:
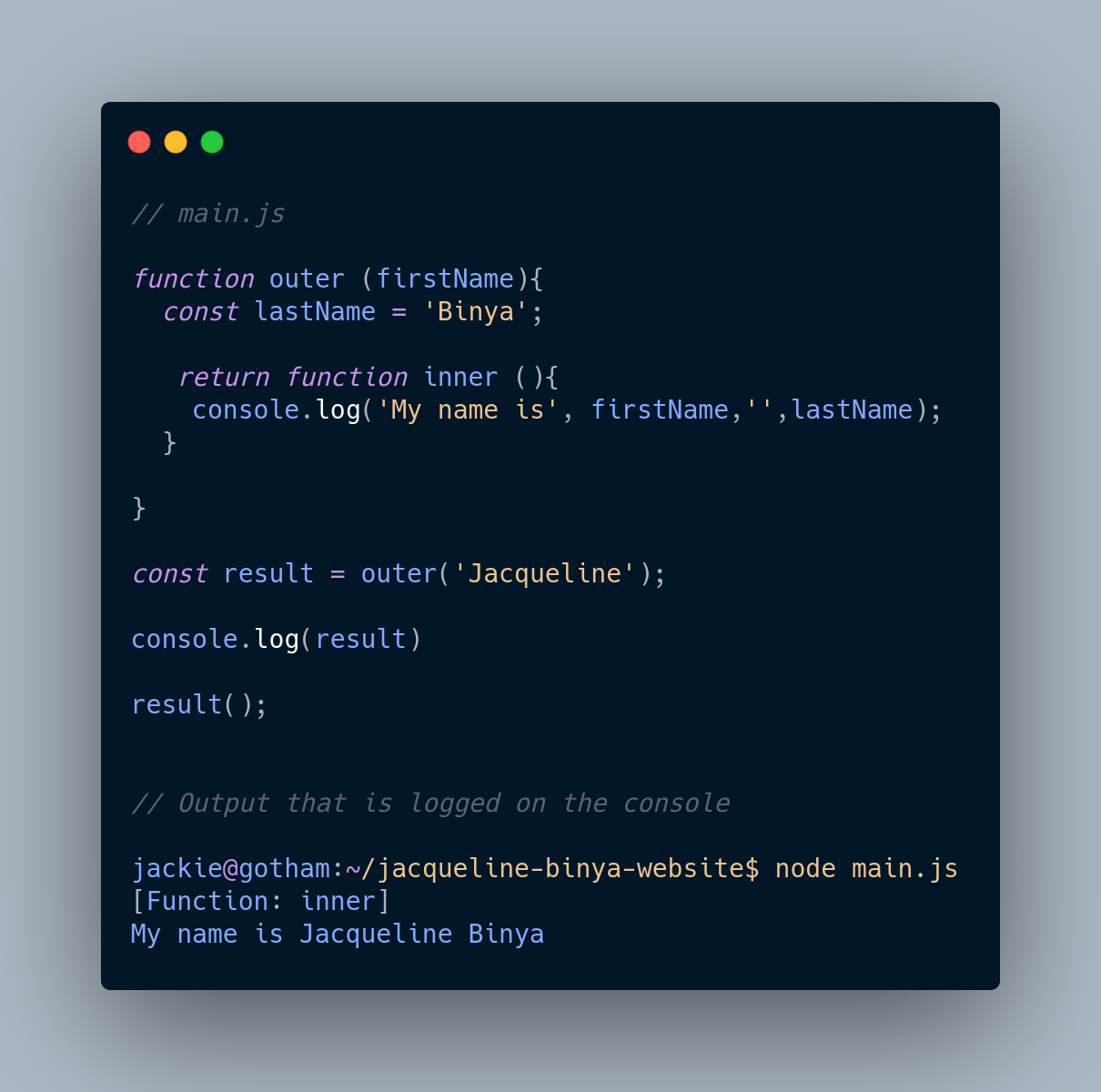
So, we have seen that when the inner function (which is a closure) is invoked its execution context’s contains a reference to the outer function’s variables, despite the fact that the outer function has already been returned.
It is my hope that this article has helped you understand closures in javascript.
Happy Coding ๐!!!